How to read a file on the classpath in Java
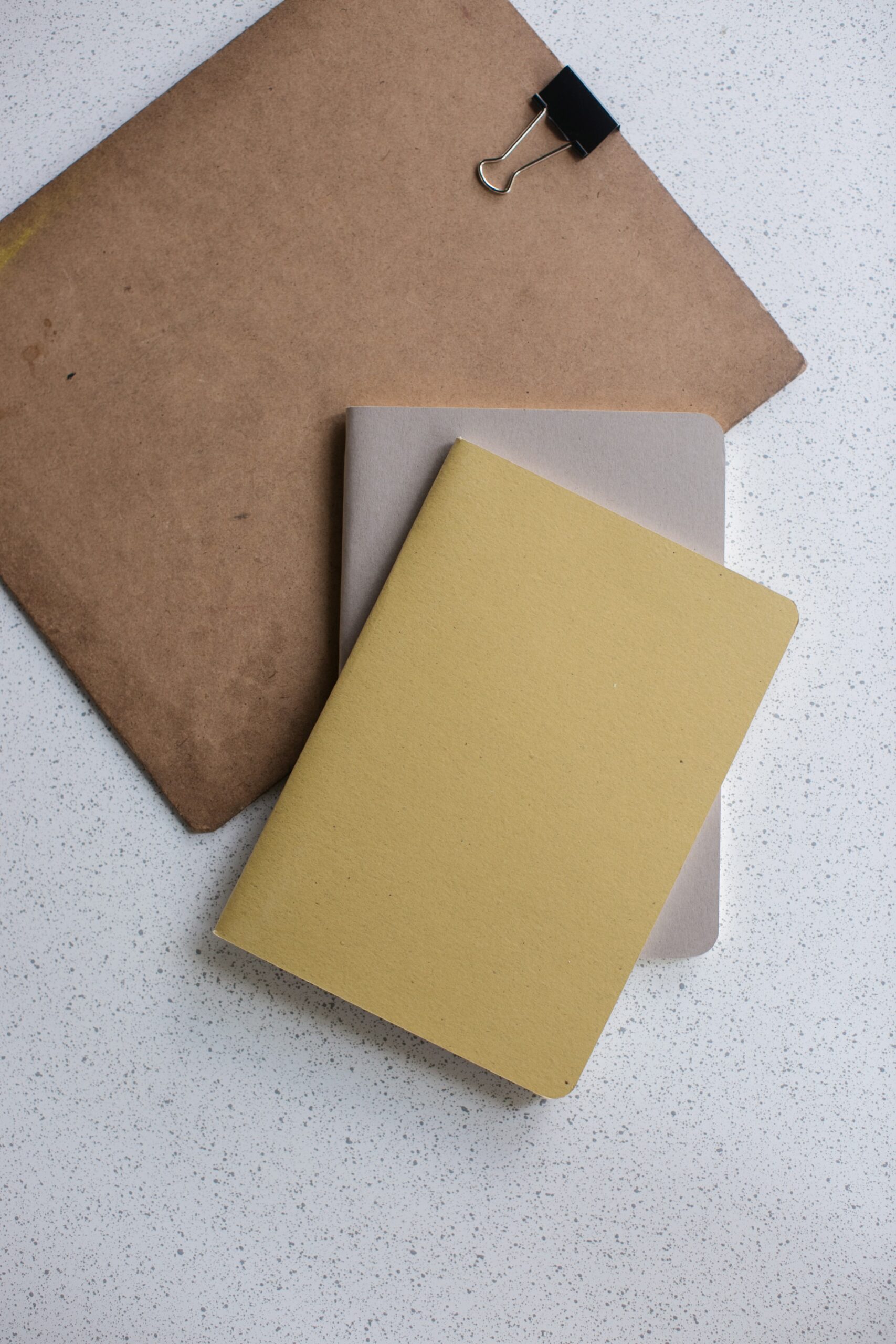
There are often programming situations when you need to read a file on the classpath via Java. In this article, I will show you how to write code for this.
Table Of Contents
Reading a file on the classpath
Let us first take a look at the code that you need to write to read a file on the classpath.
public class ReadFileFromClassPathDemo { public static void main(String[] args) throws IOException { // read in the file from the resources directory ClassLoader classLoader = ReadFileFromClassPathDemo.class.getClassLoader(); URL url = classLoader.getResource("input.txt"); String fileName = url.getFile(); File file = new File(fileName); String str = new String(Files.readAllBytes(file.toPath())); System.out.println(str); } }
Java has an in-built class called ClassLoader
. It is used to dynamically load classes. It can also be used to load resources from the classpath. The code above first obtains a ClassLoader
. It then invokes the getResource
method on the classLoader
. This loads the resource (in this case a file named input.txt) from the file system and returns an URL
instance. The URL
class has a getFile
method that returns the fully qualified filename. A File
object is then created using the filename. Finally, the Files.readAllBytes
method is used to then read the contents of the file into a String.
Running code in Eclipse
In case you need to test the code above from an IDE like Eclipse, you need to do the following:
Step 1 – Right-click on the class name and click Run As > Run Configurations:
Step 2 – Click on the Dependencies Tab:
Step 3 – Click on Classpath entries. Click on Advanced > Add External Folder:
Step 4 – Navigate to the file system and select the folder which contains the file to be read (D:/Data/Temp in my case):
Step 5 – Verify that the folder is added to the classpath. Click on Apply and Run:
Step 6 – Verify that the file is read and its contents are displayed on the console:
Maven project
In the case of a Maven project, anything placed within the src/main/resources folder of the Maven project is automatically available on the classpath. So, if you place your input file in the src/main/resources folder, the code above will be able to read it.
Further Reading
Conclusion
So, in this article, we saw how to read a file on the classpath via Java. We also saw how to run the code in Eclipse.
Also, if you'd like to test your Java knowledge, do check out my practice tests course here