Java 17 Features
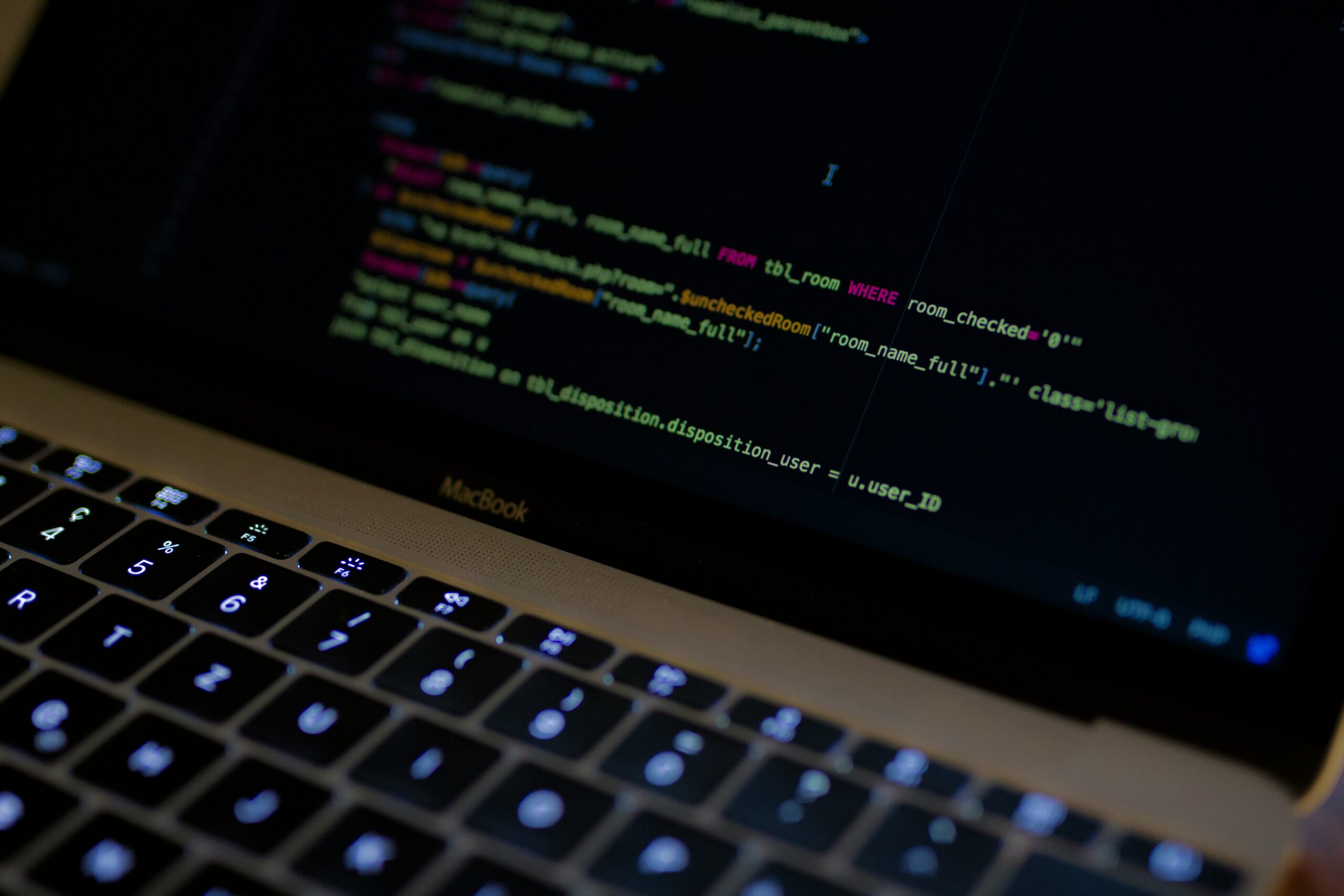
Exploring Java 17 Features
Java 17, released in September 2021, is a Long-Term Support (LTS) version, making it a significant release for developers. This version introduces several new features, enhancements, and deprecations aimed at improving the language’s performance, security, and developer productivity. Let’s explore some of the most notable features and changes in Java 17.
Pattern Matching for switch
(Preview)
Pattern matching for switch
statements simplifies complex conditional logic. This feature allows you to use patterns in switch
cases, enabling more readable and maintainable code.
switch (object) { case String s -> System.out.println("It's a string: " + s); case Integer i -> System.out.println("It's an integer: " + i); default -> System.out.println("Unknown type"); }
Sealed Classes
Sealed classes provide more control over class hierarchies. A sealed class restricts which other classes or interfaces may extend or implement it. This feature enhances code security and maintainability by limiting inheritance.
public sealed class Shape permits Circle, Rectangle, Square { } public final class Circle extends Shape { // Implementation }
New java.util.random
Interface
Java 17 introduces a new RandomGenerator
interface, providing a common API for random number generation. This interface includes methods for generating different types of random values, improving the flexibility and usability of random number generators.
RandomGenerator generator = RandomGenerator.of("L64X128MixRandom"); int randomInt = generator.nextInt();
Deprecation of the Applet API
The Applet API is officially deprecated in Java 17. This change encourages developers to move away from outdated applet technology and adopt modern alternatives like JavaFX.
Enhanced Pseudo-Random Number Generators
Java 17 introduces several new pseudo-random number generator (PRNG) algorithms, such as LXM and Xoshiro/Xoroshiro. These algorithms provide better performance and statistical properties compared to the legacy Random
class.
RandomGenerator generator = RandomGeneratorFactory.of("Xoshiro256PlusPlus").create(); long randomLong = generator.nextLong();
Strong Encapsulation for JDK Internals
Java 17 strengthens the encapsulation of JDK internals, further restricting access to non-critical internal APIs. This change promotes better application security and stability.
Removal of RMI Activation
The RMI Activation mechanism is removed in Java 17 due to its limited use and better alternatives available. This change simplifies the RMI API and encourages the adoption of modern approaches for remote method invocation.
Conclusion
Java 17 brings several exciting features and enhancements that improve the language’s usability, performance, and security. From pattern matching for switch
and sealed classes to enhanced random number generators and the deprecation of outdated APIs, this release is designed to make Java development more efficient and enjoyable. As an LTS release, Java 17 will be supported for several years, making it an excellent choice for production environments.
Also, if you'd like to test your Java knowledge, do check out my practice tests course here